Note
This page was generated from Notebooks/flopy3_drain_return.ipynb.
Flopy Drain Return (DRT) capabilities
[1]:
import sys
import os
from tempfile import TemporaryDirectory
import numpy as np
import matplotlib as mpl
import matplotlib.pyplot as plt
# run installed version of flopy or add local path
try:
import flopy
except:
fpth = os.path.abspath(os.path.join("..", ".."))
sys.path.append(fpth)
import flopy
print(sys.version)
print("numpy version: {}".format(np.__version__))
print("matplotlib version: {}".format(mpl.__version__))
print("flopy version: {}".format(flopy.__version__))
3.10.10 | packaged by conda-forge | (main, Mar 24 2023, 20:08:06) [GCC 11.3.0]
numpy version: 1.24.3
matplotlib version: 3.7.1
flopy version: 3.3.7
[2]:
# temporary directory
temp_dir = TemporaryDirectory()
modelpth = temp_dir.name
# creat the model package
m = flopy.modflow.Modflow(
"drt_test",
model_ws=modelpth,
exe_name="mfnwt",
version="mfnwt",
)
d = flopy.modflow.ModflowDis(
m,
nlay=1,
nrow=10,
ncol=10,
nper=1,
perlen=1,
top=10,
botm=0,
steady=True,
)
b = flopy.modflow.ModflowBas(m, strt=10, ibound=1)
u = flopy.modflow.ModflowUpw(m, hk=10)
n = flopy.modflow.ModflowNwt(m)
o = flopy.modflow.ModflowOc(m)
[3]:
# create the drt package
spd = []
for i in range(m.nrow):
spd.append([0, i, m.ncol - 1, 5.0, 50.0, 1, 1, 1, 1.0])
d = flopy.modflow.ModflowDrt(m, stress_period_data={0: spd})
[4]:
# run the drt model
m.write_input()
success, buff = m.run_model(silent=True, report=True)
if success:
for line in buff:
print(line)
else:
raise ValueError("Failed to run.")
MODFLOW-NWT-SWR1
U.S. GEOLOGICAL SURVEY MODULAR FINITE-DIFFERENCE GROUNDWATER-FLOW MODEL
WITH NEWTON FORMULATION
Version 1.3.0 07/01/2022
BASED ON MODFLOW-2005 Version 1.12.0 02/03/2017
SWR1 Version 1.05.0 03/10/2022
Using NAME file: drt_test.nam
Run start date and time (yyyy/mm/dd hh:mm:ss): 2023/05/04 15:59:35
Solving: Stress period: 1 Time step: 1 Groundwater-Flow Eqn.
Run end date and time (yyyy/mm/dd hh:mm:ss): 2023/05/04 15:59:35
Elapsed run time: 0.002 Seconds
Normal termination of simulation
[5]:
# plot heads for the drt model
hds = flopy.utils.HeadFile(os.path.join(m.model_ws, m.name + ".hds"))
hds.plot(colorbar=True)
[5]:
<Axes: title={'center': 'data Layer 1'}>
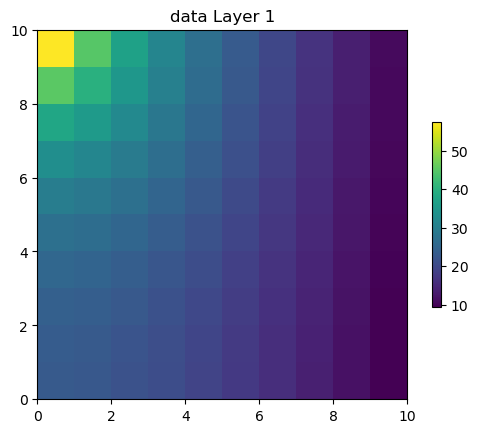
[6]:
# remove the drt package and create a standard drain file
spd = []
for i in range(m.nrow):
spd.append([0, i, m.ncol - 1, 5.0, 1.0])
m.remove_package("DRT")
d = flopy.modflow.ModflowDrn(m, stress_period_data={0: spd})
[7]:
# run the drain model
m.write_input()
success, buff = m.run_model(silent=True, report=True)
if success:
for line in buff:
print(line)
else:
raise ValueError("Failed to run.")
MODFLOW-NWT-SWR1
U.S. GEOLOGICAL SURVEY MODULAR FINITE-DIFFERENCE GROUNDWATER-FLOW MODEL
WITH NEWTON FORMULATION
Version 1.3.0 07/01/2022
BASED ON MODFLOW-2005 Version 1.12.0 02/03/2017
SWR1 Version 1.05.0 03/10/2022
Using NAME file: drt_test.nam
Run start date and time (yyyy/mm/dd hh:mm:ss): 2023/05/04 15:59:36
Solving: Stress period: 1 Time step: 1 Groundwater-Flow Eqn.
Run end date and time (yyyy/mm/dd hh:mm:ss): 2023/05/04 15:59:36
Elapsed run time: 0.002 Seconds
Normal termination of simulation
[8]:
# plot the heads for the model with the drain
hds = flopy.utils.HeadFile(os.path.join(m.model_ws, m.name + ".hds"))
hds.plot(colorbar=True)
[8]:
<Axes: title={'center': 'data Layer 1'}>
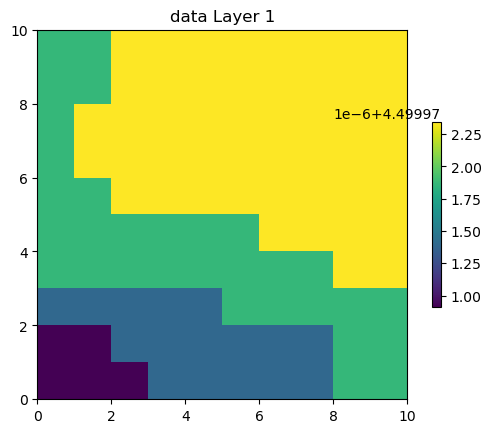
[9]:
try:
# ignore PermissionError on Windows
temp_dir.cleanup()
except:
pass